Iโm Learning Python part 2
I’m Learning Python (part 2)
<p>
<img title="Python Logo" src="http://www.python.org/images/python-logo.gif" alt="Python Logo" />
</p>
Who Uses Python?
In the world there are more than 1 million Python user, that’s because it’s
open source, and because it is implemented for every platform, and it comes
included within Linux distributions and Macintosh computers and more.
-
Google uses Python in its web search system, and it employs the Python’s
creator. - YouTube is largely written in Python.
- BitTorrent file sharing system is a Python program.
-
Intel, Cisco, HP, Seagate, Qualcomm and IB< use Python for hardware
testing. -
Industrial Light & Magic, Pixar, and others use Python in the production
of movie animation. -
NASA, Los Alamos, Fermilab, JPL, and others use Python for scientific
programming. - iRobot uses Python to develop commercial robotic vacuum cleaners ๐
- NSA uses Python for cryptography and intelligence analysis.
See Python website for more.
How Does Python work?
Python is not compiled (almost), it is interpreted. So when you run the program
the interpreter will run it line by line without compilation.
You might say “Why did you say almost?”, the answer is, Python as described
before can be compiled into byte code, so it’ll be faster for the interpreter
to execute it than the source code, just like Java’s byte code and .NET’s MSIL.
Python files have a .py extension normally, so when you write Python code you
should save it with a .py extension.
Let’s try and write our first Hello World Python program:
- Open the editor you use (Emacs, Notepad, Notepad++, โฆ).
- Write this line inside it:
- print “Hello World, It’s My First Python Program”
- Save the file as .py file.
-
Now you should tell the Python interpreter to run your file, depending
on your platform you should have the Python interpreter in (“C:\Python[VER]\”
in Windows where [VER] is the version of the Python release you have [24,
25, 26,โฆ]) or in (“/usr/bin/” in Linux). -
In Windows open a command prompt and guide it to the location of your
Python. - In Linux open a terminal and go to the location of your Python interpreter.
- Now you can execute the following command on both platforms:python [FILE]Where [File] is the location of your .py file.
- Now you should see some thing like this:python [FILE]Hello World, It’s My First Python Program
Very easy, right?
Although it is not that big program but you can run your files this way :).
Now let’s understand what happened:
The Python interpreter was given a file to run.
If it has write privileges it’ll compile it into byte code and you’ll see that
a new file was created next to your file with a .pyc extension, if it doesn’t
it’ll run it as source code.
It reads the first line (which is a print statement) and runs it and you see
the result, the execution takes place inside the PVM โ Python Virtual Machine,
which handles the execution of Python byte code and source code to run on more
than one platform without change in code.
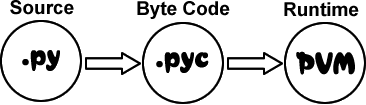
If you have a background in C or C++ you might find a change in the way of running
and compiling code, ‘cuz there is no making and linking in Python, and it is
not compiled into machine code (binary file), instead it runs inside a virtual
machine, that’s why some Python code will run faster in C++ and C.
But don’t be afraid, 90% of the time you’ll be running your programs as if they
were written in C or C++, there won’t be any difference in the time of execution,
and thatโs because of the Python simplicity which makes the program in Python
shorter and simpler than C or C++, and because it’s optimization which we’ll
describe later.
In Python you’ll notice that the compiler and the execution environment are
one thing, the compiler always present in runtime, and that leads to rapid development
cycle, with no linking and making stage.
CPython runs inside the PVM, while Jython runs inside the JVM and IronPython
.NET runs inside .NET Framework or Mono.
Jython and IronPython .NET allows the Python program to use Java and .NET classes
respectively.
If you don’t wanna use Java or .NET classes you should use CPython, ‘cuz it’s
faster and the most complete, CPython runs like C and C++ so it is the fastest.
In Jython the Python byte code is translated into Java byte code to be run inside
the JVM, while in .NET it is translated to MSIL.
What Are The Frozen Binaries?
Python has something called Frozen Binaries, which are the executables, if you
chose to create a frozen binary for your program, you’d end up with an executable
that runs on a specified platform (exe for Windows).
This executable has a great feature; it includes your program along with its
dependencies and the Python interpreter. Yes you don’t have to tell the user
to install a Python interpreter to be able to use your program, he/she doesn’t
have to know that your program is a Python program.
You can use Py2Exe to get an exe of your program (Which runs only in Windows),
or PyInstaller which gives you the ability to create a frozen binary for Windows,
Linux or UNIX. And you can use Freeze the original one. Those are available
free of charge, you can check Python website or Vaults of Parnassus (http://www.vex.net/parnassus).
What IDE’s To Use With Python?
Python comes with a simple IDE called IDLE you can use it, or you can use Emacs, but IMHO
Eclipse with PyDev make a great IDE for you and Python.
Bottom Line:
Today it was just a description on how Python runs your program, and we wrote
our Hello World program.
For the next part, try to use the ** operator – which is the power operator
in Python โ to see how much you can do in Python, e.g.:
print 2 ** 10
1024
Try huge numbers and see ๐
Cheers.